Table of content
Introduction
Ahoy, Code Adventurers! Welcome aboard our coding ship as we set sail into Lesson 3 of the Learning Series - 1.If you haven't already, take a moment to explore the valuable lessons we've covered before. You'll find them conveniently listed below for your review.
Our journey today takes us through the vast sea of Python operators, where each symbol acts as a compass guiding us to new shores of functionality.Just as sailors navigate the seas, let's explore Python operators with determination.
So, let's get started mates.
Operators
In the bustling world of Python, operators are the tireless workers, tirelessly performing tasks on our variables and values. Picture them as the backstage crew of a grand performance, meticulously adjusting the stage props and orchestrating the scenes to perfection. From adding numbers to comparing values, these operators ensure that every action on our variables and values is executed seamlessly.
They are the tools we use to perform arithmetic operations, compare values, and execute logical operations.
In the bustling metropolis of Python programming, operators come in all shapes and sizes, each with its own unique role to play in the grand scheme of code.Let's explore the different types that play crucial roles in Python programming.

Arithmetic Operators – The Math Magicians:
These operators are the wizards of arithmetic, conjuring up spells of addition, subtraction, multiplication, and division. They're like mathematicians with capes, performing complex calculations with the flick of a wand. They are mainly used for numeric values.
+ | Addition |
- | Subtraction |
* | Multiplication |
% | Modulus |
/ | Division |
// | Floor Division |
** | Exponentiation |
In Python, the '/' operator is known as "classic division" or "float division". When used, it performs division and always returns a quotient with a float data type. This means that even if the input operands are integers, the result will still be a float. Regardless of the sign of the input operands, the output will always be a float.
On the other hand, the '//' operator is referred to as "true division" or "floor division". When applied, it carries out integer division, rounding the quotient to the closest whole number. This means that the output of the '//' operator will be an integer, representing the whole number quotient of the division.
Look at this code snippet
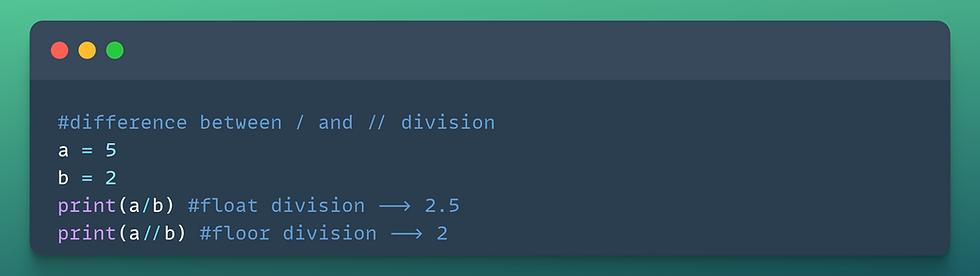
You can try it for yourself by clicking here.
Comparison Operators – The Truth Seekers:
Like detectives searching for clues, comparison operators swift through data to uncover truths. They're the sleuths of the Python world, determining if values are equal, not equal, greater than, or less than each other. Here, it can be numeric, strings and boolean.
== | Equal |
!= | Not equal |
< | Lesser than |
> | Greater than |
<= | Lesser than or equal to |
>= | Greater than or equal to |
Logical Operators – The Decision Makers:
Logical operators are the gatekeepers of logic, making decisions based on truth or falsehood. They're like bouncers at a club, allowing entry only to those who meet the criteria set by the code. They serve as the glue that binds conditional statements together in Python, enabling us to create complex decision-making structures with ease.
In upcoming lessons as we will come across conditional and control loops, this idea of 'allowing entry' will be clear.
and | Returns True if both statements are true |
or | Returns False if both statements are false |
not | Returns False if result is true and vice-versa |
Assignment Operators – The Taskmasters:
These operators are the taskmasters, assigning values to variables with authority. They're like supervisors on a construction site, directing the flow of data to where it's needed most.
They serve as the means by which values are assigned to variables in Python. They provide a straightforward and efficient way to store data in memory for later use. In Python, assignment operators are like keys to unlocking the potential of variables. It's as simple as writing a name on a label and sticking it onto a box. With the "=" sign as your trusty tool, you can effortlessly assign values to variables, transforming them into containers ready to hold whatever data you desire.
=, +=, -=, /=, %=, //=, **=, &=, |=, ^=, <<=, >>= |
Imagine the assignment operator as a versatile craftsman, not content with just the simplicity of "=" but eager to explore new horizons. Introducing the compound assignment operators. Like a team of synchronized dancers, they combine the power of a binary operator with the finesse of the simple assignment operator to perform their magic.
Picture this: two operators joining forces, executing their operation on both operands with precision and flair. Then, like a maestro conducting a symphony, they store the result into the left operand.
As you start coding, you will get used to such practices in Python.
Bitwise Operators – The Binary Boffins:
For those who dare to venture into the binary realm, bitwise operators await. They're like translators converting data into binary code, manipulating bits with precision and finesse.
In the realm of Python programming, bitwise operators are the silent ninjas, stealthily performing intricate calculations on integers. Picture them as digital acrobats, effortlessly flipping between 0s and 1s in binary form, manipulating each bit or pair of bits with precision and finesse.
When we unleash these operators upon integers, they work their magic by converting them into binary form, where each bit becomes a battleground for computation. Whether it's AND, OR, XOR, or shifting operations, these operators dance gracefully through the binary digits, crafting the result with mathematical elegance.
Once the bitwise ballet is complete, the result emerges from the shadows, transformed back into decimal format, ready to be wielded by Python programmers like a digital katana.
Bitwise operators work only on the integers.
Symbol | Name | Description |
& | AND | Sets each bit to 1 if both bits are 1 |
| | OR | Sets each bit to 1 if one of two bits is 1 |
^ | XOR | Sets each bit to 1 if only one of the two bits is 1 |
~ | NOT | Inverts all the bits |
>> | Zero fill left shift | pushes bits to the left, adding zeros from the right and dropping the leftmost bits. |
<< | Signed right shift | copies the leftmost bit to the right, filling gaps, and drops the rightmost bits. |
While bitwise operators may not be the stars of the show, particularly in the realm of machine learning where our focus lies, they still hold a fascinating allure worth exploring. If you're curious to uncover the intricacies of these operators in greater detail, venture forth to the link provided below.
Membership Operators – The Inclusion Inspectors:
Picture them as gatekeepers at an exclusive event, scrutinizing guest lists to verify if a sequence has secured its invitation to the party. With their keen eye for detail, these operators inspect each element, determining whether it's a valued member or merely an outsider looking in.
Like skilled detectives, they navigate through lists, tuples, and sets, (these are other data types which we shall soon explore in detail) uncovering the truth behind each inclusion with precision and finesse. So, as we traverse the digital landscape, let's pay homage to these diligent sentinels of sequence integrity, for without them, our data would be adrift in a sea of uncertainty.
They basically check whether a variable/expression is present in the object or not.
in | Returns True if a sequence with the specified value is present in the object |
not in | Returns True if a sequence with the specified value is not present in the object |
Identity Operators – The Unique Identifiers:
Identity operators in Python act as meticulous inspectors, examining objects at their core. Unlike other operators that focus on surface comparisons, identity operators delve deeper, probing the inner workings of objects to determine if they share the same memory location. Think of them as diligent detectives, scrutinizing each object's origins rather than their outward appearance. In the realm of Python's memory, these operators provide clarity by distinguishing true object identity from mere similarity.
is | Returns True if both variables are the same object |
is not | Returns True if both variables are not the same object |
1. Unary operators: They operate on a single operand. They perform actions such as negation, incrementation, or negation. For example, the unary minus (-) operator negates a value, turning a positive number into its negative counterpart.
2. Binary operators : They operate on two operands.They are ubiquitous in Python, handling arithmetic operations like addition, subtraction, multiplication, and division. For instance, the addition operator (+) combines two values to produce a sum.
Precedence of operators
Operator precedence is like the choreography that guides the dance of computation. Picture it as a grand ballroom, where each operator awaits its turn to take center stage. At the forefront of this elegant affair stands the exponentiation operator, adorned with the highest precedence, ready to dazzle with its powerful transformations. As the curtains rise, the floor division operator gracefully follows suit, stepping into the spotlight with poise and precision.
The rule of precedence ensures that each operator knows its place in the sequence of operations.
It's like a well-organized ensemble where every musician understands their role and timing. Just as a conductor directs the orchestra, the rule of precedence directs the flow of operations, orchestrating a harmonious performance where each operator plays its part with precision and clarity. So, as we compose our code, let us follow the lead of precedence, allowing it to guide us through the intricate melodies of Python programming with confidence and grace.
( ' ** ' has highest precedence..)
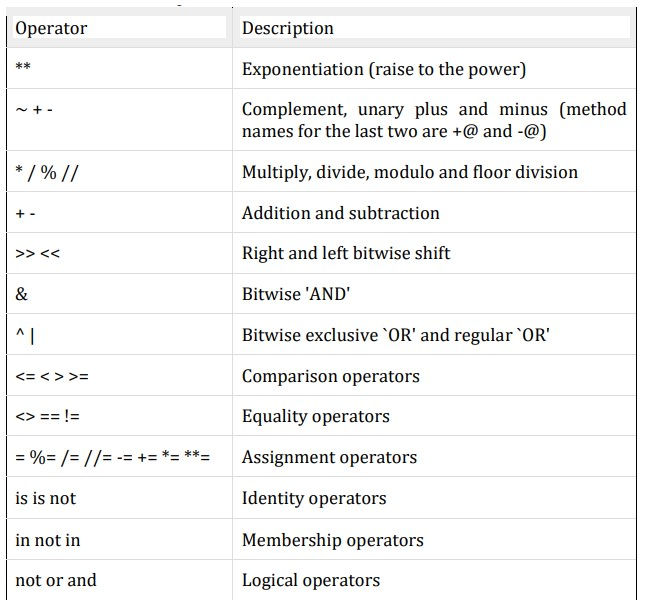
Now it's your turn to practice and play around few examples
Conclusion:
As we conclude our voyage through the dynamic realm of operators, let us reflect on the lessons learned amidst the waves of Pythonic exploration. Like seasoned sailors navigating the vast ocean of code, we have uncovered the treasures of arithmetic, comparison, logical, and other operators, each a guiding star in our quest for computational mastery.
In our next adventure, we shall embark upon the turbulent seas of control loops, where the winds of iteration and decision-making shall propel us forward toward undiscovered shores. Just as a ship relies on its sails to harness the power of the wind, we shall harness the power of control loops to steer our course through the currents of code.
So, hoist the sails, fellow adventurers, for the next leg of our journey promises excitement, discovery, and perhaps even hidden treasures waiting to be unearthed in the depths of Python's vast ocean. May the stars guide our path and the winds fill our sails as we set forth on our continuing quest for knowledge and enlightenment. Until the next tide of knowledge, stay buoyant, keep coding, and never let your learning spirit be cast adrift! Fair winds and following seas, my friends!
Link for previous lesson
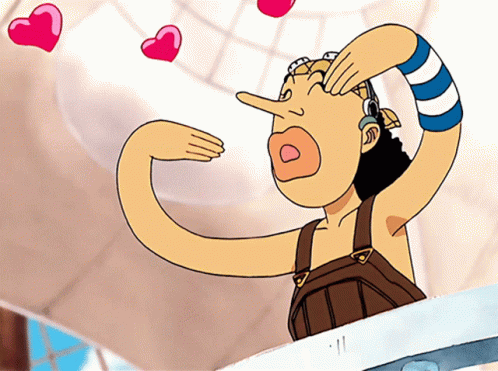
How did you like the content?
Loved it
Good
Can be improved
Comments