Table of content
Introduction
Ahoy, fellow adventurers, and welcome aboard our Python voyage! Prepare to embark on a thrilling journey through the high seas of coding as we set sail into Lesson 4 of the Learning Series - 1. If you haven't already, take a moment to explore the valuable lessons we've covered before. You'll find them conveniently listed below for your review.
In this exhilarating adventure, we'll unlock the secrets of conditional statements and loop control, guiding our ship through treacherous waters and uncharted territories. Just as skilled navigators rely on their maps and compasses to chart their course, we'll harness the power of Python's conditional statements and loop control to steer our code towards success. So hoist the sails, trim the mast, and let's navigate the Python seas together.
Conditional Statements
Like skilled captains navigating stormy seas, we must understand the power of conditional execution to steer our code toward success.
In the dynamic arena of conditional statements, each condition takes on the role of a valiant knight, jostling for position in the quest for truth. Like noble warriors in a grand tournament, they advance one by one, wielding their boolean swords with precision and determination. If the first knight falters in their quest, the next bravely steps forward, undeterred by the challenge ahead.
Yet, amidst this epic struggle, only one knight can emerge victorious, the first to pierce the veil of falsehood and claim victory for their cause. Even if others stand ready, their blades honed and hearts resolute, they must yield to the champion who first vanquishes the enemy of doubt. In the arena of Python's code, it's not just about being true, but about embodying the spirit of courage and perseverance as we navigate the labyrinth of conditional logic.
Now Python supports the same logical conditions i.e, the logical operators (know more)
if statement
It is a fundamental component of programming languages. It serves as a conditional statement, allowing the programmer to check a condition and execute a specific block of code if the condition evaluates to true. In essence, the if statement acts as a gatekeeper, determining whether certain actions should be taken based on the current state of the program.
By using if statements, programmers can introduce decision-making capabilities into their code, enabling it to respond dynamically to different situations. This allows for the creation of more flexible and responsive programs that can adapt to varying conditions during execution.
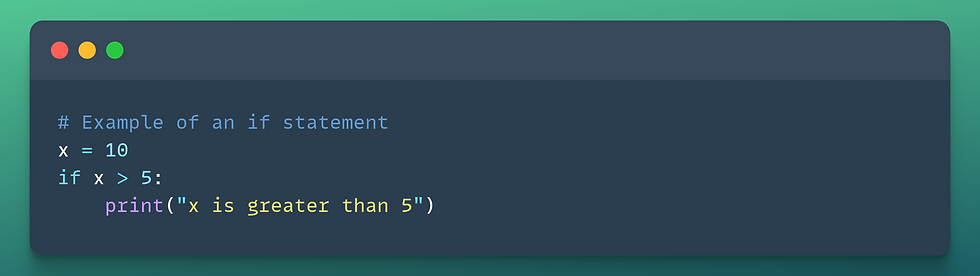
So here, we have a variable `x` assigned the value of 10. The 'if statement' checks if the value of `x` is greater than 5. If the condition evaluates to True, the indented block of code under the if statement is executed. Since the value of `x` (10) is indeed greater than 5, the message "x is greater than 5" is printed to the console. If the condition were False, the indented code block under the if statement would be skipped.
Have you wondered the importance of Boolean expressions? Why use them only?
Well, they control the flow of execution, enable dynamic behavior, and facilitate error handling. In essence, boolean expressions are fundamental tools for creating responsive and intelligent programs.
Did you know? Boolean expressions are named after mathematician George Boole, who first developed the concepts of boolean algebra in the 19th century. Talk about leaving a lasting legacy in the world of programming!
Extending the decision-making process in Python goes beyond simple if statements. Enter elif and else clauses, the dynamic duo of conditional execution!
Elif statement
The elif clause in Python expands our decision-making capabilities by enabling us to evaluate multiple conditions in sequence. It serves as a bridge between the initial if statement and the final else clause, offering a middle ground for exploring alternative scenarios.
When the if statement evaluates to false, the elif clause steps in, providing an opportunity to examine additional conditions. This sequential evaluation allows for a more nuanced approach to decision-making, where each condition builds upon the previous one.
Think of elif as a series of checkpoints along a journey. If the first checkpoint is missed, we move on to the next one, and so forth, until we reach our destination or exhaust all options. Each elif condition acts as a waypoint, guiding the flow of execution based on the current state of affairs.
Else statement
And then there's the else clause, the loyal companion that swoops in when all other conditions fail. When none of the preceding if or elif conditions evaluate to true, the else clause steps in to provide a default course of action. It's the "just in case" option, offering reassurance that our program won't encounter unexpected behavior or errors due to unanticipated conditions.
The else clause offers peace of mind and stability, serving as the fail-safe mechanism that guarantees our code will always have a plan B. It's the guardian angel of conditional statements, ready to swoop in and rescue our program from the brink of uncertainty.
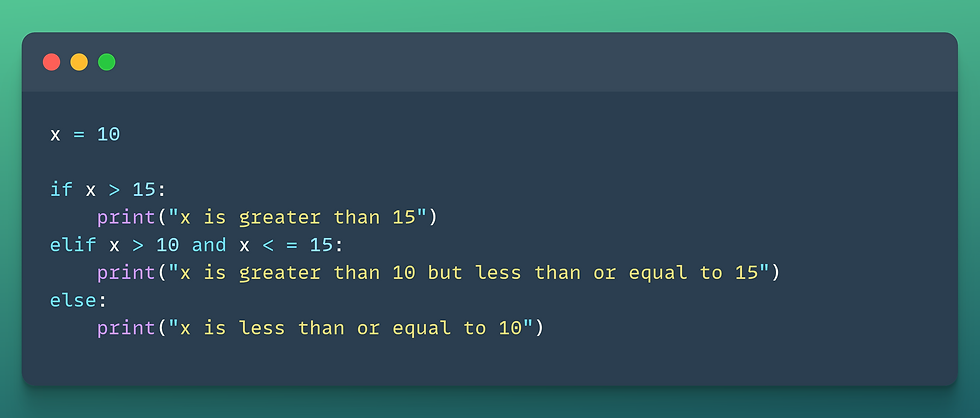
In this example, we have a variable `x` assigned the value of 10. The 'if statement' checks if the value of `x` is greater than 15. If the condition is True, the first indented block of code under the 'if statement' is executed. If the condition is False, the elif statement is evaluated. Here, the elif statement checks if `x` is greater than 10. If True, the corresponding indented block of code under elif is executed. If both the if and elif conditions are False, the else statement's block of code is executed.
Handling multiple conditions and fallback scenarios in Python involves a strategic approach to conditional logic. By combining if, elif, and else clauses, we can construct a robust decision-making framework that accommodates various scenarios.
'if' statements evaluate the primary condition. If true, the corresponding code executes; otherwise, elif clauses provide alternative paths. Each elif acts as a backup plan, handling multiple scenarios. If none match, the else clause executes, providing a default action.
Allowing us to create flexible and resilient code that can respond effectively to a variety of situations.
Conditional expressions in Python, also known as ternary expressions, provide a concise way to write conditional statements in a single line of code. It offer a compact and readable way to incorporate conditional logic into Python code, especially when the condition and results are simple expressions. However, they should be used judiciously to maintain code clarity and readability.
Example for Conditional expressions
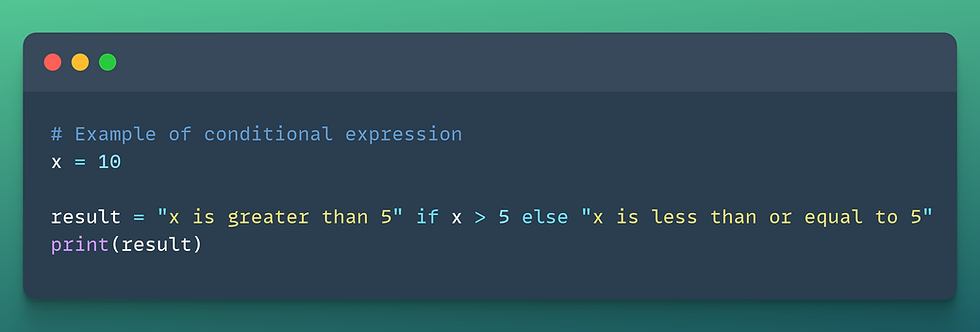
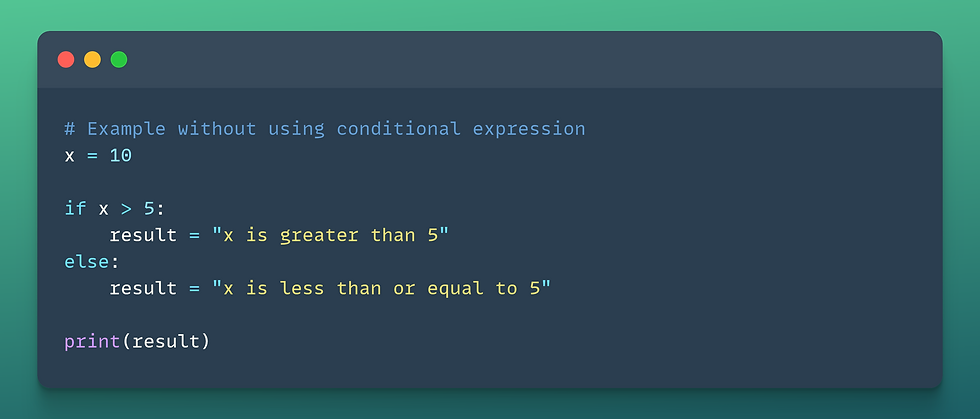
In the first snippet, we use a conditional expression to assign the value of `result`. The syntax of a conditional expression in Python is `value_if_true if condition, else value_if_false`. Here, the condition is `x > 5`. If the condition is True, the value "x is greater than 5" is assigned to `result`. If the condition is False, the value "x is less than or equal to 5" is assigned to `result`.
Control Loops
So what are control loops?
Control loops are the backbone of programming, enabling repetitive execution of tasks with ease. Picture them as the rhythm section of a musical composition, setting the beat for the code to follow. Essentially, control loops allow us to automate processes by repeating a set of instructions multiple times until a certain condition is met.
Imagine you're a treasure hunter exploring a mysterious island. To uncover hidden riches, you dig through layers of sand, one shovel at a time. Each shovel represents a step in the process, and you continue digging until you strike gold or until you've searched the entire area. This repetitive digging process mirrors the concept of a control loop in programming.
Now let us understand loops as control flow mechanism
Consider a treasure hunter meticulously searching a deserted island, scouring every inch of sand for buried treasure. With a loop, they can methodically explore each square foot of the beach, continuing their search until they unearth the coveted chest of riches. The mechanism makes it lot easier to move in sequence.
Whether we're processing large datasets, generating complex patterns, or simply counting from one to a hundred, loops offer a flexible and efficient means of controlling the flow of our code. They empower us to tackle a wide range of problems with precision and finesse, transforming our programs into dynamic and responsive creations. So, as we embark on our journey through the realm of Python programming, let us embrace the rhythmic cadence of loops, harnessing their power to navigate the twists and turns of our digital adventures with confidence and creativity.
'for' loop
A control flow statement used for iterating over a sequence of elements. It systematically traverses each item in the sequence (here, sequence is referred as lists, tuples and dictionary which will be discussed in upcoming lessons), executing a block of code for each iteration. The syntax of a for loop typically consists of the keyword "for" followed by a variable name that represents each item in the sequence, the keyword "in",and then the sequence itself.
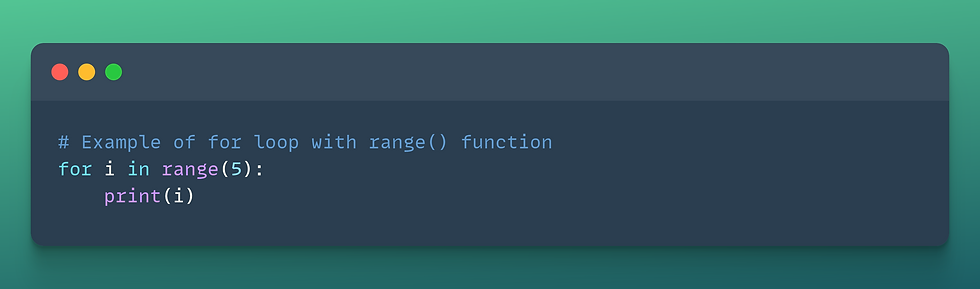
This code snippet iterates through numbers from 0 to 4 using a for loop. Each number is assigned to the variable 'i', and then printed to the console. Therefore, it will print numbers 0, 1, 2, 3, and 4, each on a new line.
'while' loop
A control flow statement used for executing a block of code repeatedly as long as a specified condition is true. The syntax of a while loop consists of the keyword "while" followed by a condition, which is evaluated before each iteration of the loop. If the condition evaluates to True, the code block inside the loop is executed. This process continues until the condition becomes False.
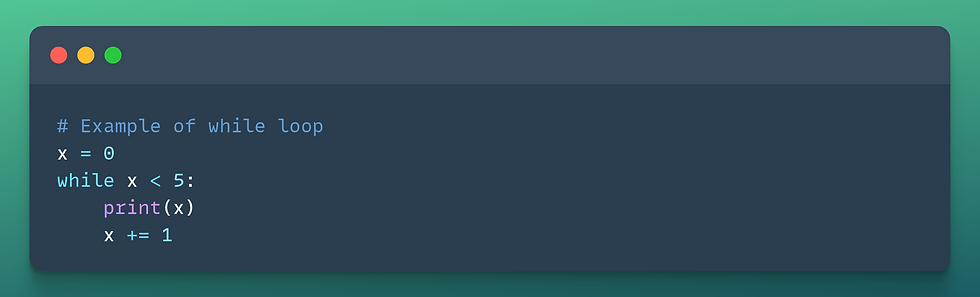
This code initializes a variable 'x' to 0 and enters a while loop. While 'x' is less than 5, the value of 'x' is printed to the console, and then incremented by 1 in each iteration. Therefore, it prints numbers 0, 1, 2, 3, and 4, each on a new line.
Some other useful statements
Break statement
Ah, the break statement – a swift and decisive tool in the arsenal of Python programmers. Picture yourself navigating through a dense forest, with each step requiring careful consideration. Suddenly, you stumble upon a hidden path, but as you venture forth, you encounter an unexpected obstacle.
In this moment, the break statement becomes your trusted companion. With a single command, you can halt your journey through the forest, regardless of how far you've traveled. It's like discovering a secret exit that allows you to escape the maze at any moment. And here it is the loop statements.
Continue statement
Picture this: as you traverse through the forest, you stumble upon a tangled thicket of brambles. Instead of getting entangled in the maze of prickly branches, you gracefully sidestep them with the continue statement, seamlessly moving past the obstruction and continuing on your path.
Similarly, in the realm of Python programming, the continue statement empowers you to navigate through loops with ease and agility. When faced with undesirable elements or error-prone iterations, the continue statement allows you to gracefully skip over them, keeping your code flow uninterrupted and your progress unhindered.
Pass statement
Picture it as a blank canvas awaiting the stroke of the artist's brush, a stage awaiting the actor's performance, or a ship docked at port, waiting to set sail on its maiden voyage. In the vast expanse of our codebase, the pass statement stands as a placeholder for untold adventures yet to unfold.
Hahaha that was over-poetic. What I meant to say is that 'pass statement' in Python serves as a placeholder for code that will be implemented later. It is used when a syntactically required statement needs to be present, but no action or computation is intended at the moment.
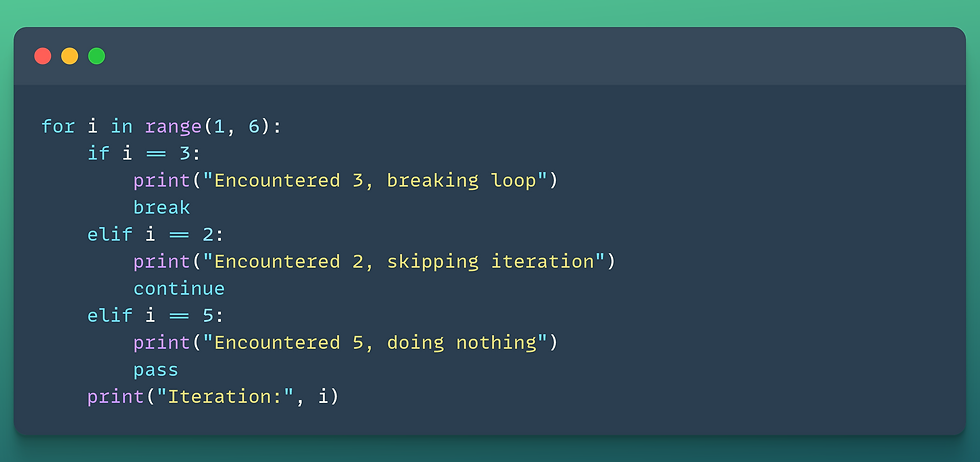
The for loop iterates over values from 1 to 5 using the range function. Inside the loop, each value of i is checked against specific conditions using if, elif, and else statements.
If i equals 3, the loop is terminated immediately using the break statement.
If i equals 2, the current iteration is skipped using the continue statement.
If i equals 5, the loop continues without executing any specific action (using the pass statement).
After each conditional check, the value of i and a corresponding message are printed to the console. Finally, the loop iterates through all values from 1 to 5, executing the appropriate actions based on the conditions specified.
Nested Loop
These are loops that are placed within other loops. They allow you to iterate over a sequence of items multiple times, with each iteration of the outer loop triggering a complete iteration of the inner loop.
Look at this snippet below

The outer loop iterates over values from 1 to 3 using the range function. Inside the outer loop, an inner loop iterates over values from 1 to 3 using another range function.
Within the inner loop, conditional statements are used to control the flow of execution. If the inner loop iteration is 2, the current iteration is skipped using the continue statement.
If the outer loop iteration is 3 and the inner loop iteration is 3, the inner loop is terminated using the break statement.
After each conditional check, messages indicating the iteration of both the outer and inner loops are printed to the console. Finally, the nested loops iterate through all combinations of values from 1 to 3, executing the appropriate actions based on the conditions specified.
Ah, it seems the time has come for me to flex my virtual muscles and embark on a journey of coding exploration! Like a captain setting sail on a new adventure, I shall navigate through the vast sea of Pythonic possibilities, charting a course through the waves of conditional statements, loops, and control flow.
Conclusion
As the sun sets on this week's coding escapade, it's time to bid adieu to our current topics and set our sights on the horizon of new adventures. Join us next week as we delve into the fascinating world of Lists – a cornerstone of Python programming that unlocks endless possibilities for data manipulation and organization.
Get ready to embark on a journey through the realm of lists, where we'll uncover the secrets of creating, accessing, and manipulating data structures with finesse and flair. From traversing through elements to unleashing the power of list comprehensions, there's no limit to what we can achieve with lists by our side.
So, fellow adventurers, sharpen your pencils, charge your laptops, and prepare to dive headfirst into the realm of lists. Until then, may your code be as dynamic as the changing tides, and your programming prowess as vast as the open sea.
Until next time, happy coding, and may the Pythonic spirit guide you on your coding endeavors. Farewell for now, and see you soon on our next thrilling voyage through the world of Python programming. Keep Learning, never be wasted!!!
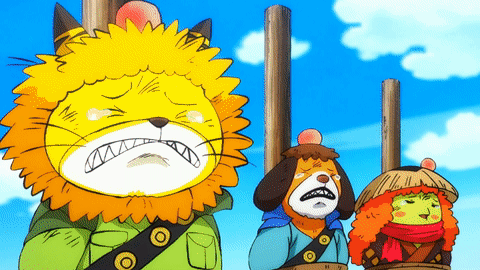
How did you like the content?
Loved it
Good
Can be improved
Comentários